[51. HUD (Heads Up Display) #1]
HUD는 화면에 표시되는 정보의 모든 것이다. (체력바, 보유재화 등등)
HUD의 변수들을 넣을 클래스는 개발에 따라 다르다. 그 중 한가지 방법은 모든 HUD의 항목을 플레이어 컨트롤러에 두는 것이다.
게임 도중 캐릭터가 죽으면 캐릭터가 사라졌다가 다시 생성될수는 있겠지만 플레이어 컨트롤러는 그대로 유지된다.
만약 정보가 캐릭터에 귀속 되어있다면, 캐릭터가 사망시 모든 정보가 초기화되기 때문에 캐릭터에서 관리할 수 없다.
UMG를 사용하여 HUD를 구현할 것이기 때문에 UMG(Unreal Motion Graphic) 모듈을 프로젝트에 포함시켜야 한다.
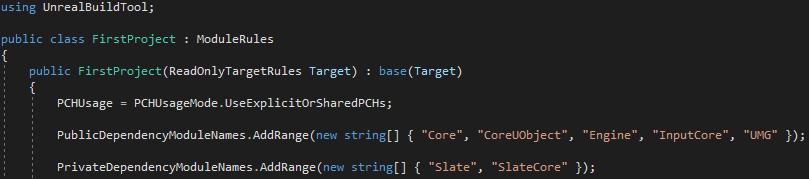
'프로젝트명.cs' 에서 추가시켜주면 된다.
이제 위젯 블루프린트를 이용해서 UI를 만들어 보려고 한다.
다른 모든 위젯들을 포함하는 전체 위젯, 즉 HUD 오버레이가 될 것이기 때문에 HUDOverlay로 이름을 짓는다.
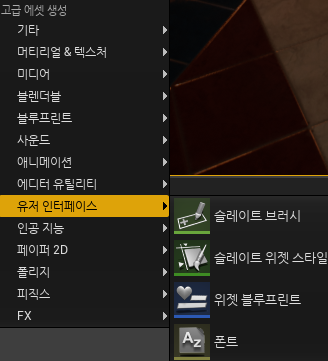
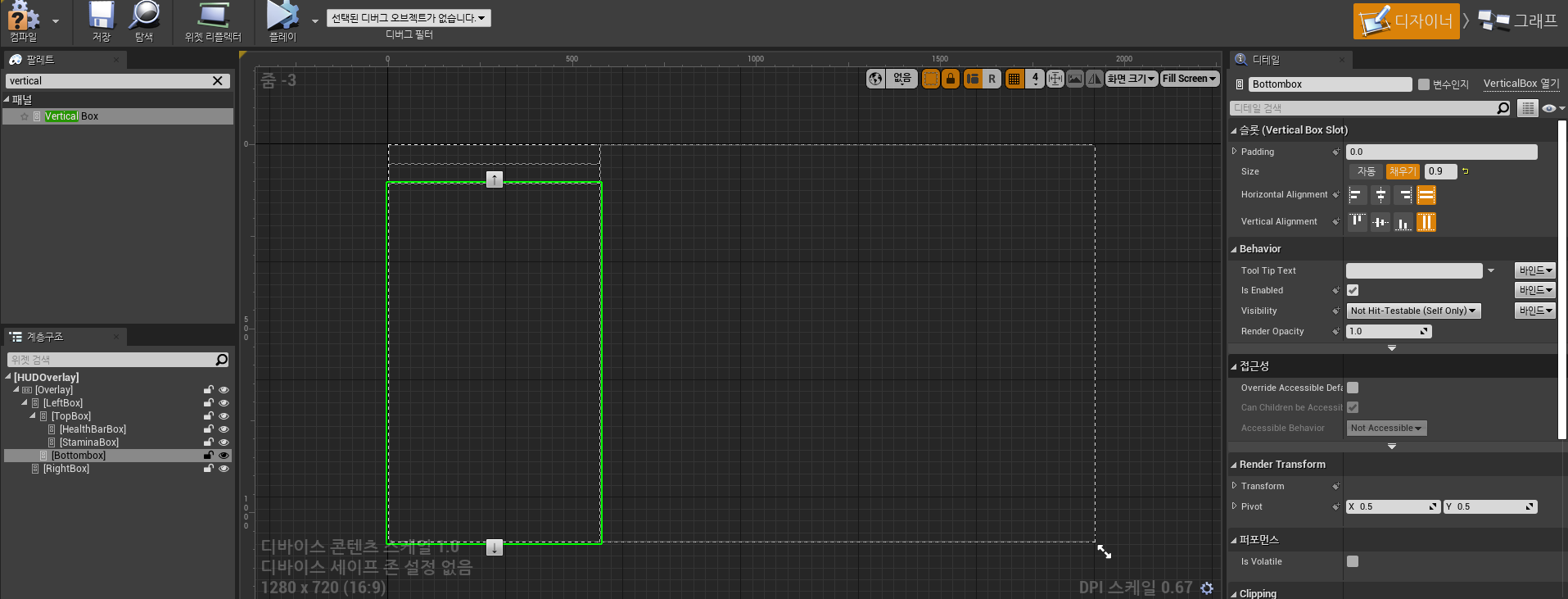
Box들을 이용해서 각 영역의 비율을 정해줄 수 있다. 디테일 탭의 채우기와 비율을 이용하면 된다.
계층구조에 따라 상대적으로 영역을 분할한다.

Padding값 설정으로 간격을 줄 수 있다.
체력바를 만들어 보자.
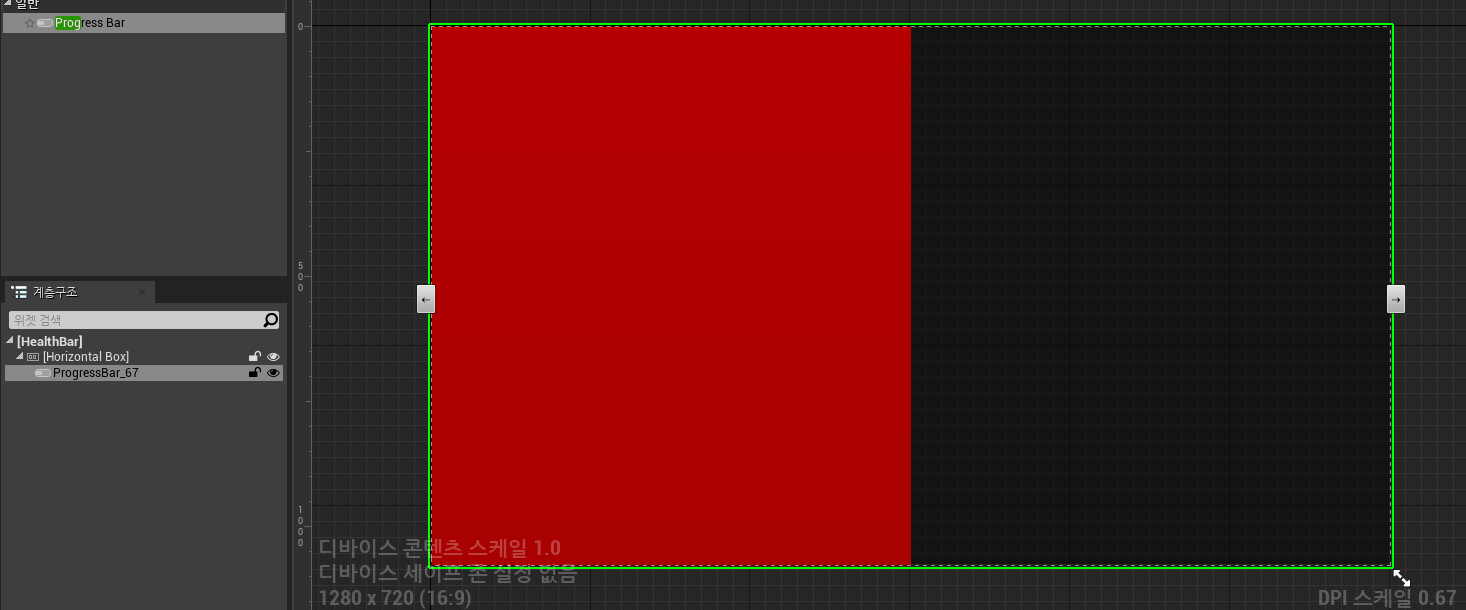
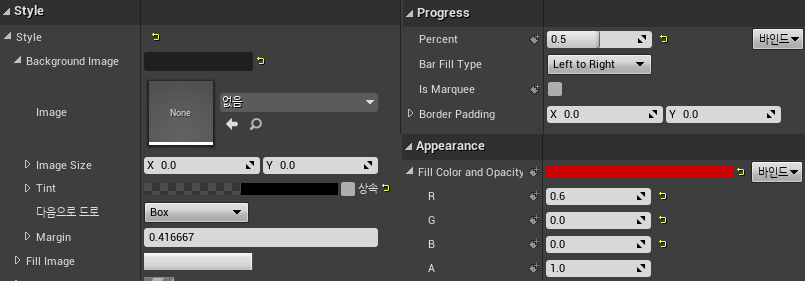
Style은 Progress의 Percent가 감소되었을 때 표시되는 영역이다. 이미지나 틴트로 변화를 줄 수 있다.
Appearance는 Percent의 비율만큼 채워진 영역이다.
다 만들었으므로 HUDOverlay에 배치해볼 시간이다.
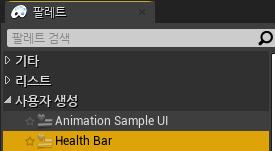
사용자 생성(User Created) 탭에 방금 만든 위젯이 나온다.
HealthBarBox의 하위 계층에 넣어주면 된다.
[52. HUD #2]
C++ 코드와 HUD를 연동시켜보자.
PlayerController를 상속받는 MainPlayerController C++ 클래스를 만든다.
// MainPlayerController.h
public:
/* Reference to the UMG asset in the editor */
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category="Widgets")
TSubclassOf<class UUserWidget> HUDOverlayAsset;
/* Variable to hold the widget after creating it */
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category="Widgets")
UUserWidget* HUDOverlay;
protected:
virtual void BeginPlay() override;
// MainPlayerController.cpp
#include "Blueprint/UserWidget.h"
void AMainPlayerController::BeginPlay()
{
Super::BeginPlay();
if (HUDOverlayAsset)
{
HUDOverlay = CreateWidget<UUserWidget>(this, HUDOverlayAsset);
}
HUDOverlay->AddToViewport(); // 뷰포트에 생성
HUDOverlay->SetVisibility(ESlateVisibility::Visible); // HUD Hiding?
}
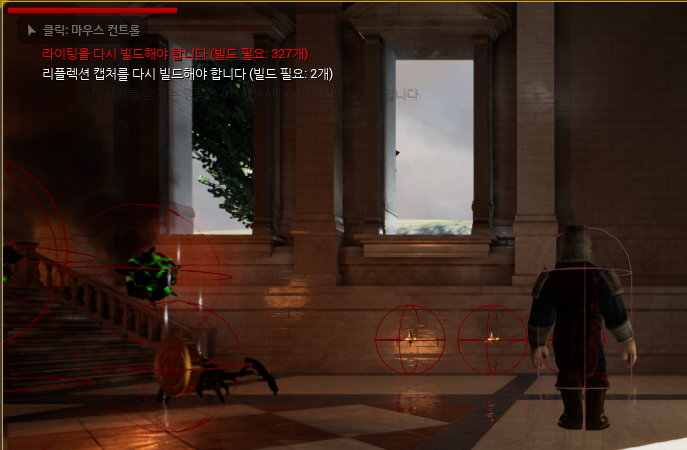
조그맣지만 좌상단에 잘 표시된다.
[53. HUD #3]
우선은 블루프린트를 이용해서 체력을 연동시켜 보자.
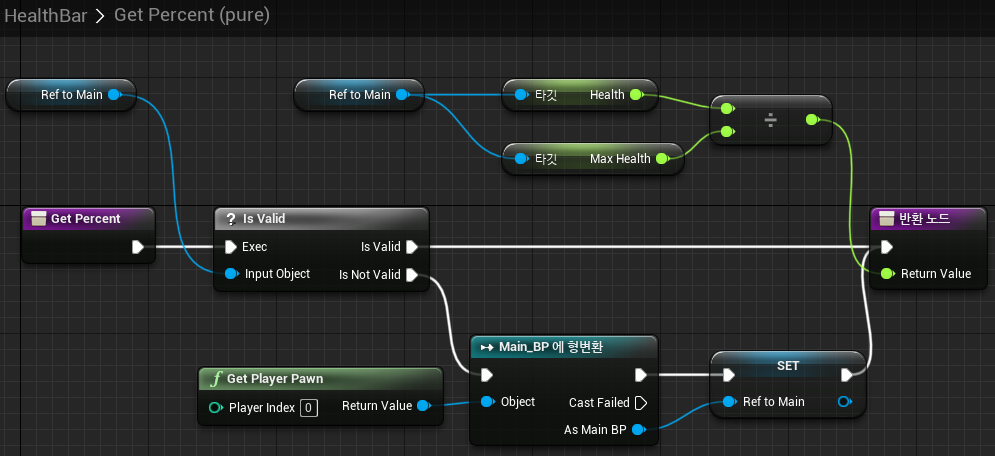
HealthBar의 Percent를 바인딩 해준다.
// Main.h
public:
UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category="Player Stats")
float MaxHealth;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Player Stats")
float Health;
UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category = "Player Stats")
float MaxStamina;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Player Stats")
float Stamina;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Player Stats")
int32 Coins;
// Main.cpp
AMain::AMain()
{
MaxHealth = 100.f;
Health = 65.f;
MaxStamina = 350.f;
Stamina = 120.f;
Coins = 0;
}
코드까지 작성해주면 바인딩은 끝난다. 하지만 실시간으로 체력이 변화하는걸 확인할 수단이 없으므로 프로토 타입으로 간단히 만들어서 확인해본다.
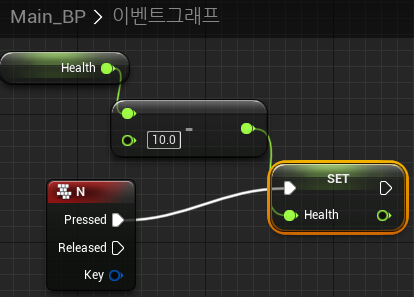
N키를 누르면 10씩 감소된다. 실행해보면 실제로 잘 적용된다.
스태미너도 똑같이 만들어주면 동일하게 잘 적용된다.
[54. HUD #4]
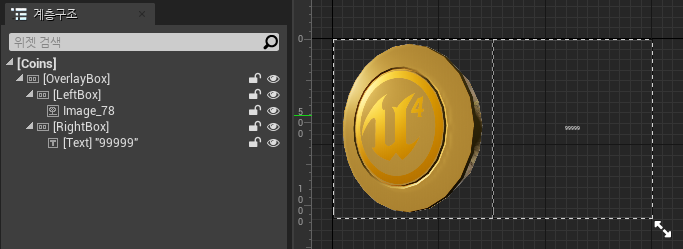
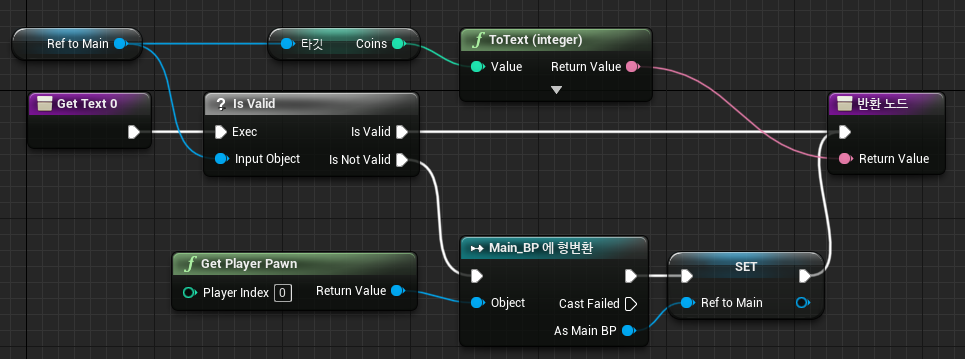
위젯 블루프린트를 만들고 Text에 기존과 같이 바인딩을 해주면 된다.
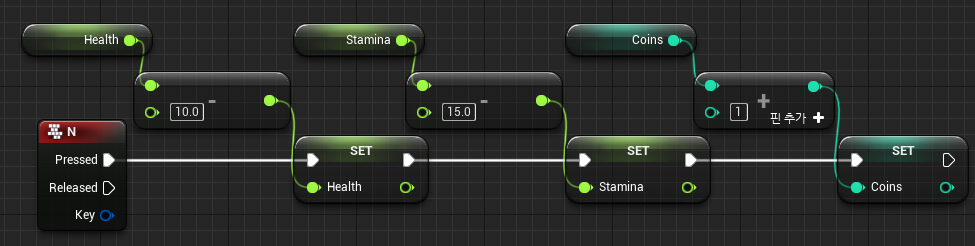
코인까지 연결시켜주면 N키 한번에 값이 변경되고 UI에도 잘 적용된다.
[정리]
- UI를 구성하기 위한 프레임 워크에는 UMG, HUD, Slate가 있다. 보통 UMG를 이용한다. 슬레이트를 감싼 프레임워크가 UMG라고 보면 된다. 조금 더 로우레벨이기 때문에 오로지 코드만으로 UI을 구현하고 싶다면 슬레이트로 만들 수 있다.
'언리얼 엔진 > UE4 C++' 카테고리의 다른 글
[UE C++] Gameplay Mechanics #7 (0) | 2022.09.09 |
---|---|
[UE C++] Gameplay Mechanics #6 (0) | 2022.09.09 |
[UE C++] Gameplay Mechanics #4 (0) | 2022.09.08 |
[UE C++] Gameplay Mechanics #3 (0) | 2022.09.07 |
[UE C++] Gameplay Mechanics #2 (0) | 2022.09.07 |